Simple ReactJs Tutorial
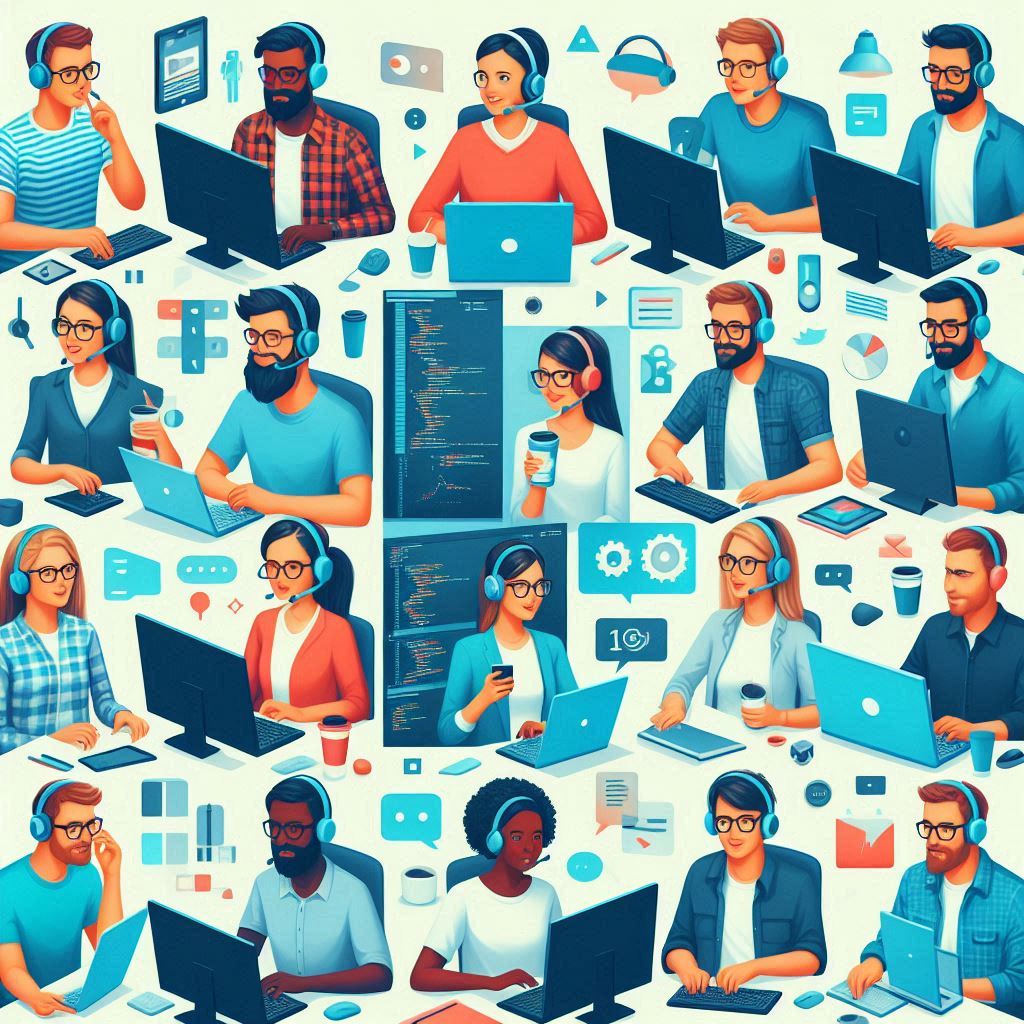
Here’s a beginner-friendly tutorial to get you started with ReactJS, a popular JavaScript library for building user interfaces, especially single-page applications.
Setting Up Your React Environment To begin, you’ll need to set up a React environment on your computer. This typically involves the following steps:
- Install Node.js: React requires Node.js to run, so make sure it’s installed on your system.
- Create a React App: Use the
create-react-app
command to set up a new React project. This command creates a new folder with all the necessary files and configurations.npx create-react-app my-react-app
- Start the Development Server: Navigate to your project directory and start the development server.
This will open a new browser window with your React application running.cd my-react-app npm start
Understanding React Basics React is all about components. A component is a JavaScript function that returns HTML (via JSX).
- JSX: JSX is a syntax extension for JavaScript that looks similar to HTML. It allows you to write HTML elements in JavaScript and place them in the DOM without any
createElement()
andappendChild()
calls. - Components: Components are the building blocks of any React application. You can create a component for each part of your UI, making your code reusable and easy to manage.
Creating a Simple Component Here’s an example of a simple React component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
Rendering a Component You can render your component to the DOM like this:
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<Welcome name="World" />);
State and Lifecycle State is a set of data that determines the component’s behavior and rendering. You can add state to your component using the useState
hook.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
Handling Events React events are named using camelCase, rather than lowercase. With JSX, you pass a function as the event handler, rather than a string.
<button onClick={activateLasers}>
Activate Lasers
</button>
Conditional Rendering In React, you can create distinct components that encapsulate behavior you need. Then, you can render only some of them, depending on the state of your application.
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
if (isLoggedIn) {
return <UserGreeting />;
}
return <GuestGreeting />;
}
Lists and Keys You can build collections of elements and include them in JSX using curly braces {}
.
const numbers = [1, 2, 3, 4, 5];
const listItems = numbers.map((number) =>
<li key={number.toString()}>
{number}
</li>
);
Forms Handling forms is about how you handle the data when it changes over time. React provides useState
to handle form inputs.
function NameForm(props) {
const [name, setName] = useState('');
const handleSubmit = (event) => {
alert('A name was submitted: ' + name);
event.preventDefault();
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={(e) => setName(e.target.value)} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
For more detailed tutorials and advanced topics, you can explore resources like W3Schools, React official documentation, TutorialsPoint, and React’s own introductory tutorial. These resources offer comprehensive guides and exercises to deepen your understanding of ReactJS.
Happy coding with ReactJS! π
Related Posts
-
-
-
-
-
AI and Web Technologies
7/3/2024 -