Building a Mobile App with Ionic Angular: A Comprehensive Guide with Sample Code
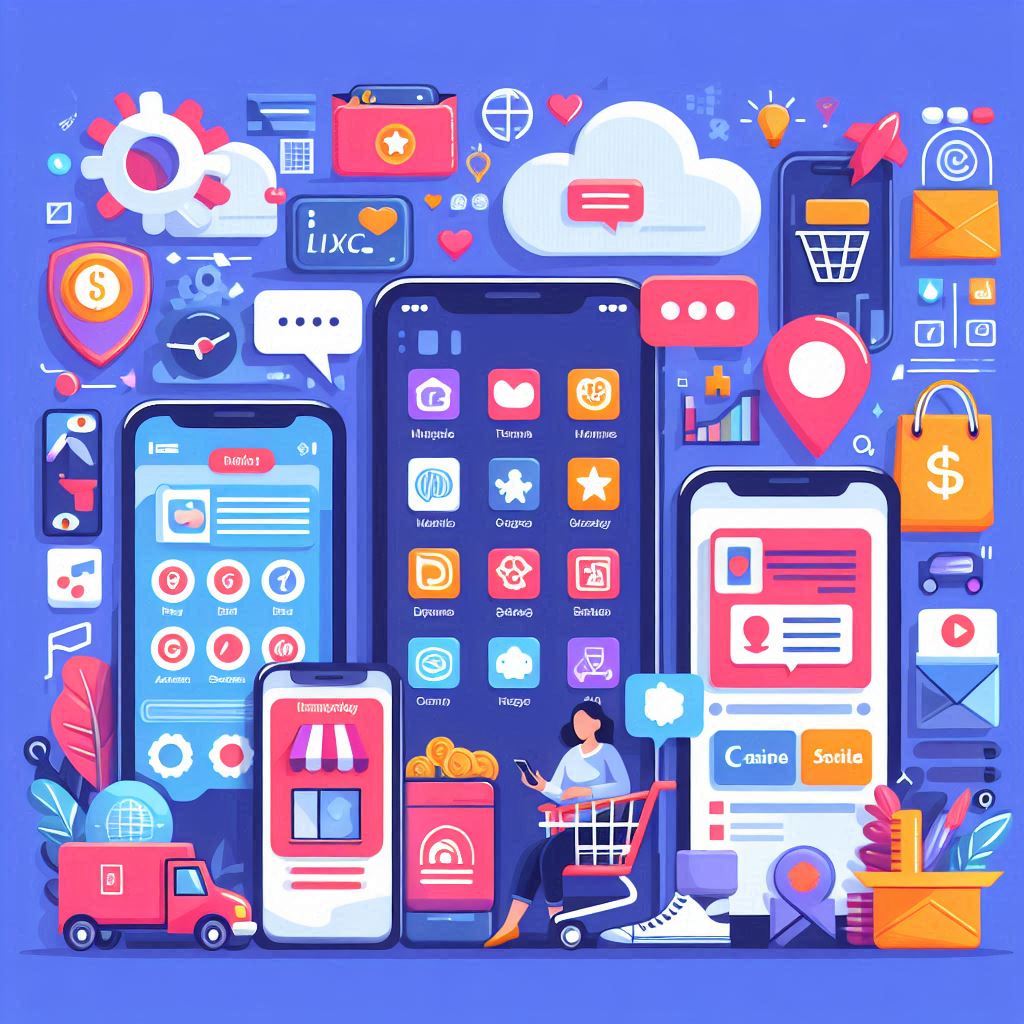
Building a mobile app with Ionic Angular is an excellent choice for developers looking to create cross-platform applications using web technologies. Ionic leverages HTML, CSS, and JavaScript to build apps that run seamlessly on both iOS and Android. This comprehensive guide will walk you through the process of building a mobile app with Ionic Angular, from setting up your development environment to deploying your app, complete with sample code.
Setting Up Your Development Environment
The first step in building a mobile app with Ionic Angular is to set up your development environment. You will need to install Node.js, which is required for running the Ionic CLI and other development tools. Once Node.js is installed, you can install the Ionic CLI globally using npm:
npm install -g @ionic/cli
The Ionic CLI is a powerful tool that simplifies the process of creating, building, and deploying Ionic apps. Additionally, you will need a code editor, such as Visual Studio Code, which provides a robust environment for writing and debugging your code.
Creating a New Ionic Project
With your development environment set up, the next step is to create a new Ionic project. The Ionic CLI makes this process straightforward. You can create a new project by running the ionic start
command followed by the name of your project and the template you want to use. For this guide, we will use the tabs template, which creates a simple app with a tab-based navigation structure:
ionic start myApp tabs --type=angular
Once your project is created, navigate to the project directory and start the development server using the ionic serve
command:
cd myApp
ionic serve
This command launches a local development server and opens your app in a web browser. The development server supports live reloading, which means that any changes you make to your code will be automatically reflected in the browser. This feature is incredibly useful for quickly iterating on your app’s design and functionality.
Building the User Interface
The next step is to build the user interface of your app. Ionic provides a rich set of UI components that you can use to create a modern and responsive interface. These components include buttons, cards, forms, and more, all of which are designed to work seamlessly across different platforms. Here is an example of how to create a simple form using Ionic components:
<ion-header>
<ion-toolbar>
<ion-title>
My App
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-card>
<ion-card-header>
<ion-card-title>Login</ion-card-title>
</ion-card-header>
<ion-card-content>
<form>
<ion-item>
<ion-label position="floating">Username</ion-label>
<ion-input type="text"></ion-input>
</ion-item>
<ion-item>
<ion-label position="floating">Password</ion-label>
<ion-input type="password"></ion-input>
</ion-item>
<ion-button expand="full" type="submit">Login</ion-button>
</form>
</ion-card-content>
</ion-card>
</ion-content>
Adding Navigation
In addition to UI components, Ionic also provides a powerful navigation system that allows you to manage the flow of your app. The tabs template includes a basic navigation structure with three tabs, each corresponding to a different page. You can add new pages to your app by generating them using the Ionic CLI. For example, you can create a new page called “Profile” by running the following command:
ionic generate page Profile
This command creates a new folder with the necessary files for the page, including an HTML template, a TypeScript file, and a stylesheet. Once you have created your pages, you can configure the navigation by updating the routing configuration in the app-routing.module.ts
file:
const routes: Routes = [
{
path: 'tabs',
component: TabsPage,
children: [
{
path: 'home',
loadChildren: () => import('./home/home.module').then(m => m.HomePageModule)
},
{
path: 'profile',
loadChildren: () => import('./profile/profile.module').then(m => m.ProfilePageModule)
},
{
path: '',
redirectTo: '/tabs/home',
pathMatch: 'full'
}
]
},
{
path: '',
redirectTo: '/tabs/home',
pathMatch: 'full'
}
];
Adding Functionality
With the user interface and navigation in place, the next step is to add functionality to your app. Ionic integrates seamlessly with Angular, a popular web framework, allowing you to leverage Angular’s powerful features and libraries. You can use Angular services to manage data, handle HTTP requests, and implement business logic. Here is an example of how to create a service to fetch data from a remote API:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class DataService {
private apiUrl = 'https://api.example.com/data';
constructor(private http: HttpClient) {}
getData(): Observable<any> {
return this.http.get<any>(this.apiUrl);
}
}
You can then use this service in your components to fetch and display data:
import { Component, OnInit } from '@angular/core';
import { DataService } from '../services/data.service';
@Component({
selector: 'app-home',
templateUrl: './home.page.html',
styleUrls: ['./home.page.scss'],
})
export class HomePage implements OnInit {
data: any;
constructor(private dataService: DataService) {}
ngOnInit() {
this.dataService.getData().subscribe(response => {
this.data = response;
});
}
}
Accessing Native Device Features
In addition to Angular, Ionic also supports Capacitor, a cross-platform runtime that allows you to access native device features using JavaScript. Capacitor provides a set of plugins for common device features, such as the camera, geolocation, and storage. You can install these plugins using npm and then use them in your app by importing the corresponding Capacitor module. Here is an example of how to use the Capacitor Camera plugin to capture photos:
npm install @capacitor/camera
import { Component } from '@angular/core';
import { Camera, CameraResultType } from '@capacitor/camera';
@Component({
selector: 'app-profile',
templateUrl: './profile.page.html',
styleUrls: ['./profile.page.scss'],
})
export class ProfilePage {
photo: string;
async takePhoto() {
const image = await Camera.getPhoto({
quality: 90,
allowEditing: false,
resultType: CameraResultType.Base64
});
this.photo = `data:image/jpeg;base64,${image.base64String}`;
}
}
Testing Your App
Once you have implemented the functionality of your app, the next step is to test it on real devices. Ionic provides several tools for testing and debugging your app, including the Ionic DevApp and the Ionic Lab. The Ionic DevApp is a mobile app that allows you to preview your Ionic app on your device without the need for a full build. You can connect your device to the same network as your development machine and use the Ionic DevApp to scan a QR code generated by the ionic serve
command.
The Ionic Lab is another powerful tool that allows you to preview your app on multiple platforms simultaneously. By running the ionic serve --lab
command, you can open a special preview mode that displays your app in both iOS and Android layouts side by side. This feature allows you to compare the appearance and behavior of your app on different platforms and make any necessary adjustments to ensure a consistent user experience.
Building and Deploying Your App
After testing your app, the final step is to build and deploy it to the app stores. Ionic uses Capacitor to build native binaries for iOS and Android. You can generate the native project files by running the ionic capacitor build
command, which creates the necessary Xcode and Android Studio projects. You can then open these projects in their respective IDEs and use the standard build and deployment tools to create the final app binaries. Once the binaries are generated, you can submit them to the Apple App Store and Google Play Store for review and distribution.
ionic capacitor build ios
ionic capacitor build android
Conclusion
Building a mobile app with Ionic Angular is a powerful and efficient way to create cross-platform applications using web technologies. By following this comprehensive guide, you can set up your development environment, create a new Ionic project, build a user interface, add functionality, test your app on real devices, and deploy it to the app stores. With Ionic’s rich set of UI components, powerful navigation system, and seamless integration with Angular and Capacitor, you can create modern and responsive mobile apps that run seamlessly on both iOS and Android. As you continue to explore and experiment with Ionic, you will discover even more features and capabilities that can help you build high-quality mobile applications.
Related Posts
-
-
-
-
-
AI and Web Technologies
7/3/2024 -